In programming, control structures are generally important in shaping the flow of code execution. Among the most critical decision-making constructs are “if-else” and “switch case” statements. These two constructs are applied to execute specific blocks of code based on different conditions or multiple possibilities. However, despite their shared objective of control flow, they differ in their application, readability and performance.
What Is A Switch Case Statement?
A switch case statement in programming is a statement that tests the value of a variable and compares it with multiple cases. In the process, when the case match has been found a block of statements associated with that given case is executed. Each case in a block of a switch has a different name/number which is known as an identifier. The value provided by the user is compared with all the cases inside the switch block until the appropriate match is found. If the appropriate case match is found then the default statement is executed and the control goes out of the switch block.
It is an alternative of “if-else” statement and is particularly useful when you have multiple conditions to check against a single variable or expression. The switch case statement improves code readability and maintainability, especially when dealing with a large number of possible cases.
Features of Switch Case
- Switch case takes decision on the basis of equality.
- Each case refers back to the original expression.
- Each case has a break statement. The default may or may not use the break statement.
- The data type that can be used in switch expression is integer type only.
- The switch case statement analyses the value of an expression and a block code is selected on the basis of that evaluated expression.
The basic structure of a switch case statement in most programming languages is as follows:
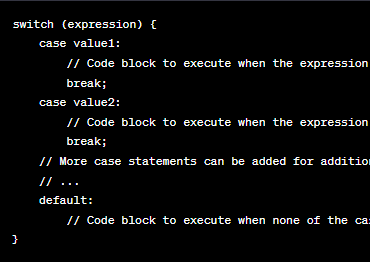
Here is how the switch case statement works:
- Expression: The expression represents the variable or value whose result will be used to determine which case to execute. It must be of a data type that can be compared, such as integers, characters, or enumerations.
- Cases: Each case represents a specific value that the expression is compared against. If the expression matches a case value, the corresponding code block associated with that case will be executed.
- Code Blocks: The code block associated with each case contains the instructions that will be executed when the corresponding case matches the expression. These code blocks should be indented under their respective case labels.
- Break Statement: After executing the code block for a matched case, a break statement is used to exit the switch block and prevent the execution of subsequent case blocks. If a break statement is not included, the program will continue executing the code blocks of all subsequent cases regardless of whether their conditions are met.
- Default Case: The optional “default” case is used to provide a code block that executes when none of the case values match the expression. It is similar to the “else” block in an “if-else” statement. If the default case is omitted and none of the cases match, the switch block will be skipped entirely.
What Is An If-else Statement?
An if-else statement in programming is a conditional statement that runs a different set of statement depending on whether an expression is true or false. The if-else statement executes a block of code if a specified condition is true. If the condition is false, another block of code can be executed.
In other words, it provides a way to make decisions in the code and choose between different courses of action depending on whether a given condition is true or false.
Features of if-else Statement
- Multiple statements are used for multiple choices.
- If statement evaluates integer, character, pointer or floating-point type or Boolean type.
- Statement will be executed depending upon the output of the expression inside if statement.
- Every time, either true block or false block will be executed.
The basic syntax of an if-else statement in most programming languages looks like this:
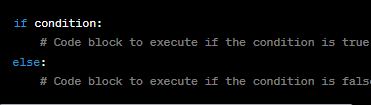
Here’s a step-by-step explanation of how an if-else statement works:
- Condition: The if-else statement starts with a condition, which is an expression that evaluates to either true or false. The condition can be a simple comparison (e.g.,
x > 10
,age == 18
) or a more complex expression involving logical operators (and
,or
,not
) and parentheses. - Evaluation: When the program encounters an if-else statement, it evaluates the condition to determine whether it is true or false.
- Execution: If the condition is true, the code block inside the
if
statement is executed. If the condition is false, the code block inside theelse
statement is executed.
Well, the else
block is optional. If there is no else
block, the program will simply skip that part and continue executing the code after the if-else statement.
Here is a Python example to illustrate the if-else statement:
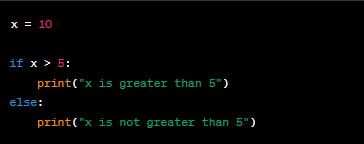
From the above example, if the value of x
is greater than 5, the first print statement will be executed, otherwise, the second one will be executed. The outcome will be “x is greater than 5” since x
is equal to 10 in this case.
Also Read: Difference Between Switch Case And Else If Ladder
Difference Between If-else And Switch Case Statement In Tabular Form
BASIS OF COMPARISON | IF-ELSE | SWITCH CASE |
Check the Testing Expression | An if-else statement can test expression based on range of values or conditions. | A switch statement tests expressions based only on a single integer, enumerated value or string object. |
Ideal for | If-else conditional branches are great for variable conditions that results into Boolean. | Switch statements are ideal for fixed data values. |
Creation of Jump Table | In if-else case we do not create a jump table and all cases are executed at runtime. | In switch case, we create jump table on compiled time only selected case is executed on runtime. |
Type of Search | If else implements linear search. | Switch implements binary switch. |
Condition & Expression | Having different conditions is not possible. | We can only have one expression. |
Evaluation | If-else statement evaluates integer, character, pointer or floating-point type or Boolean type. | Switch statement evaluates only character or integer value. |
Sequence of Execution | It is either if-statement will be executed or else-statement is executed. | Switch case statement executes one case after another till a break statement appears or until the end of switch statement is reached. |
Default Execution | If the condition inside if statements is false then by default the else statement is executed if created. | If the condition inside switch statements does not match with any of cases, for that instance, the default statement is executed if created. |
Values | Values are based on constraint. | Values are based on user choice. |
Use | It is used to evaluate a condition to be true or false. | It is used to test multiple values of the same variable or expression like 1, 2, 3 etc. |
Editing | It is difficult to edit the if-else statement, if the nested if-else statement is used. | It is easy to edit switch cases as they are recognized easily. |
Key Takeaway
Not all programming languages support the switch case statement in the same way. Some languages impose restrictions on the types of expressions and case values, while others allow more flexible conditions. Some languages allow the use of other data types and patterns in the cases, such as strings or ranges of values. Always refer to the specific language documentation to understand the exact syntax and rules for the switch case statement in that language.
Lastly, If-else statements are crucial in programming as they allow your code to respond to different scenarios based on conditions, enabling you to implement decision-making logic in your programs. You can use them in tasks like user input validation, flow control, and error handling.
Also Read: Difference Between While And Do-While