Procedural and object programming are the two approaches that form the backbone of modern software development, shaping how programmers structure and organize their code to achieve different goals. Each present a unique set of concepts, principles, and techniques that influence how developers design, implement, and maintain their codebase.
The focus of procedural programming is to break down a programming task into a collection of variables, data structures and subroutines whereas in object-oriented programming is to break down a programming task into objects that expose behavior (methods) and data (members or attributes) using interfaces. Let us talk in details how the two different approaches differ from one another.
What is Object-Oriented Programming (OOP)?
Object-Oriented Programming (OOP) is a programming paradigm that structures code around the concept of “objects.” An object is a self-contained unit that combines data (attributes or properties) and the operations (methods or functions) that can be performed on that data. OOP aims to model real-world entities, relationships, and behaviors in a more intuitive and organized manner. In other words, this model of programming, computer programs are usually designed using the concept of objects that interact with real world.
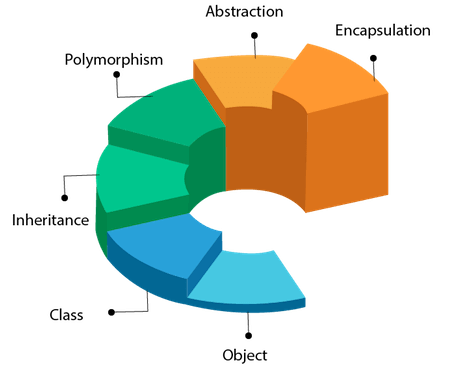
Concepts of Object-Oriented Programming
- Classes and Objects: A class is a blueprint or template for creating objects. An object is an instance of a class. Classes define the attributes (data) and methods (functions) that objects of that class will possess.
- Encapsulation: This refers to the bundling of data (attributes) and methods (functions) that operate on the data into a single unit, i.e., the object. Access to the internal data is controlled through access modifiers (public, private, protected) to ensure data integrity and security.
- Inheritance: Inheritance allows one class (subclass or derived class) to inherit attributes and methods from another class (superclass or base class). It promotes code reuse and establishes a hierarchical relationship among classes.
- Polymorphism: Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables the use of a single interface to represent different types of objects. Polymorphism is achieved through method overriding and interfaces/abstract classes.
- Abstraction: Abstraction involves simplifying complex reality by modeling classes based on essential attributes and behaviors. It hides the unnecessary details while exposing the necessary features.
There are normally many object oriented programming languages; however the most popular ones are class-based, meaning that objects are instances of classes which also determine their types.
Object-Oriented Programming provides a structured and modular approach to software development, enabling developers to create more maintainable, reusable, and scalable code. It’s widely used in various programming languages like Java, Python, C++, and C#.
Example
Let’s consider a simple example of a banking application using OOP concepts.
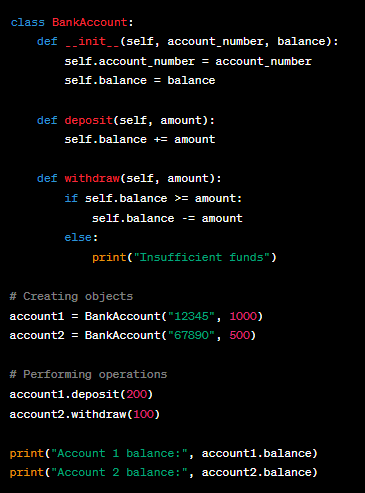
In this example, the BankAccount
class encapsulates attributes (account number, balance) and methods (deposit, withdraw) that operate on those attributes. Objects (account1
and account2
) are instances of the class, each with its own data and behavior.
What is Procedural Programming?
Procedural Programming is a programming paradigm that focuses on using procedures or functions to structure code. In this paradigm, the program is divided into a set of functions or procedures that operate on data. These functions are defined sequentially and can be executed in a linear fashion. Procedural programming is centered around the idea of executing a series of instructions step by step, often without strong data abstraction. During a program’s execution, any given procedure might be called at any point, including other procedures or itself.
Concepts of Procedural Programming
- Functions or Procedures: Functions are the building blocks of procedural programs. They encapsulate a sequence of instructions that perform a specific task. Data is passed as arguments to these functions, and the result is returned.
- Global Variables: Procedural programs often use global variables to store and manage data that can be accessed by multiple functions. This can lead to issues related to data integrity and maintainability.
- Control Structures: Procedural programming relies heavily on control structures such as loops (for, while) and conditionals (if, else) to control the flow of execution based on conditions.
- Limited Data Abstraction: Data and functions are separate in procedural programming, but there is limited support for encapsulating data and behavior together in a structured way.
Example
Let’s consider a simple procedural program that calculates the average of a list of numbers in Python:
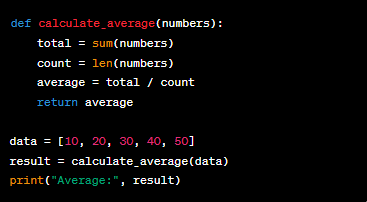
In this example, the program is structured around the calculate_average
function, which takes a list of numbers as input and returns the average. The data is stored in a global variable (data
), and the function operates on it.
Also Read: Difference Between Source Code And Object Code
Key Difference: Procedural Oriented vs Object Oriented
1. Paradigm
- Procedural Programming: Focuses on procedures/functions as the primary organizational unit.
- Object-Oriented Programming: Focuses on objects as the primary organizational unit.
Example
Procedural:
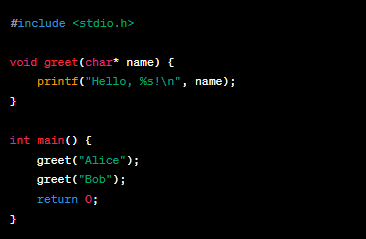
Object-Oriented:
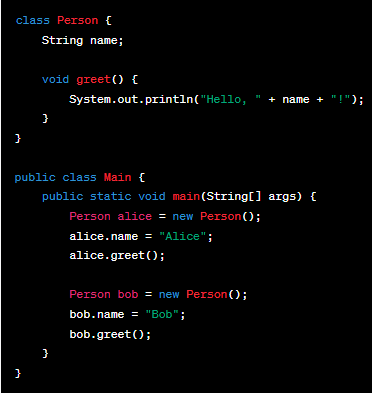
2. Abstraction
- Procedural: Limited abstraction through functions and data structures.
- OOP: High-level of abstraction through classes and objects.
Example
Procedural:
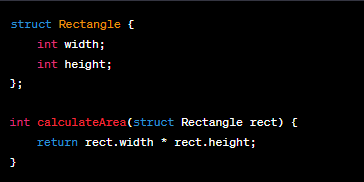
Object-Oriented:
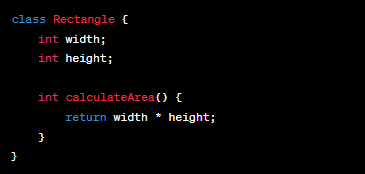
Read Further: Stack Vs. Heap In C++
3. Encapsulation
- Procedural: Limited support for encapsulation.
- OOP: Strong support for encapsulation through access modifiers (e.g., public, private).
Example:
Object-Oriented (encapsulation):
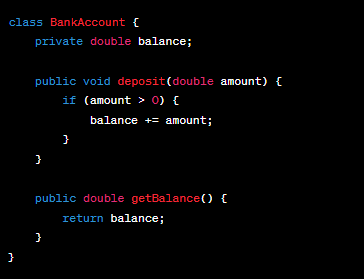
4. Inheritance
- Procedural: No inherent support for inheritance.
- OOP: Supports inheritance, allowing classes to inherit properties and methods from other classes.
Example:
Object-Oriented (inheritance):
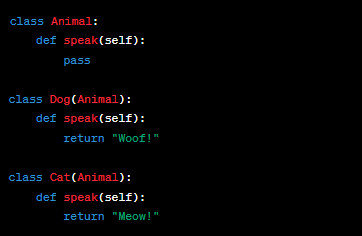
5. Polymorphism
- Procedural: Limited support for polymorphism.
- OOP: Supports polymorphism through method overriding and interfaces/abstract classes.
Example:
Object-Oriented (polymorphism):
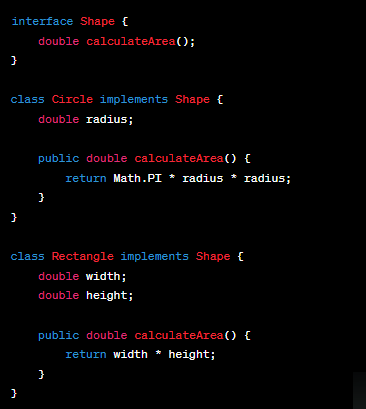
6. Code Reusability
- Procedural: Limited code reusability.
- OOP: High code reusability through inheritance and composition.
Example:
Object-Oriented (code reusability):
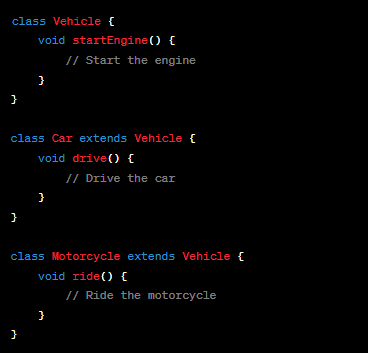
7. Data Hiding
- Procedural: Limited data hiding.
- OOP: Supports data hiding through encapsulation and access modifiers.
Example:
Object-Oriented (data hiding):
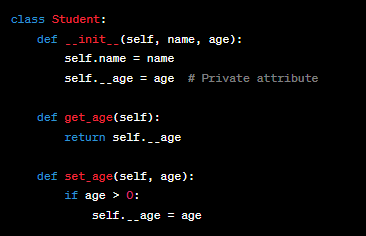
8. Message Passing
- Procedural: Emphasizes function calls.
- OOP: Emphasizes message passing between objects.
Example:
Object-Oriented (message passing):
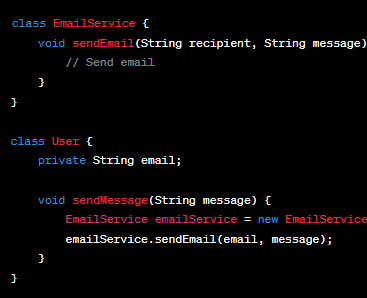
9. Focus on State
- Procedural: Focuses on the data and its manipulation.
- OOP: Focuses on both data and behavior (methods) that operate on the data.
Example:
Object-Oriented (focus on state):
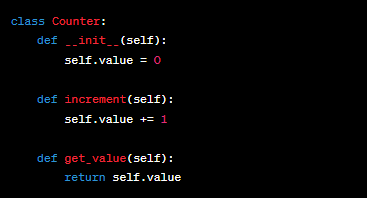
10. Code Organization
- Procedural: Tends to result in larger, harder-to-manage codebases.
- OOP: Promotes modularization and easier code organization.
11. Flexibility
- Procedural: Limited flexibility when extending functionality.
- OOP: Offers greater flexibility and extensibility.
12. Real-World Analogies
- Procedural: Resembles a recipe or set of instructions.
- OOP: Resembles a collection of interacting objects/entities.
Procedural Oriented vs Object Oriented Programming: Key Takeaway
BASIS OF COMPARISON | OBJECT ORIENTED PROGRAMMING (OOP) | PROCEDURAL ORIENTED PROGRAMMING (POP) |
Division Of Program | In object oriented programming, program is divided into parts referred to as objects. | In procedural oriented programming, program is divided into small parts referred to as functions. |
Data Movement | Objects can move and communicate with each other through member function. | Data can move freely from function to function in the system. |
Importance | Importance is given to the data rather than procedures or functions because it works as a real world. | Importance is not given to data but to functions as well as sequence of actions to be done. |
Approach | Object oriented programming follows Bottom Up approach. | Procedural oriented programming follows Top Down approach. |
Security | Objected oriented programming provides data hiding so it is more secure. | But procedural programming does not have any proper way for hiding data so it is less secure. |
Virtual Classes | Concept of virtual function appears during inheritance. | No concept of virtual classes. |
Most Important Attribute | Data is more important than function. | Function is more important than data. |
Overloading | Overloading in the form of function overloading and operator overloading is possible in object oriented programming. | In procedural oriented programming, overloading is not possible. |
Access Modes | In Object oriented programming, there are three accessing modes “public”, “private”, “protected’’ that are used as an accessing share to access attributes or functions. | In procedural oriented programming, there is no specific accessing mode to access attributes or functions in the program. |
Code Reusability | The existing code in object oriented programming can be reused by the feature referred to as inheritance. | There is no such feature in procedural oriented programming. |
Addition of New Data And Function | In object oriented programming, adding new data and function is easy. | Adding new data and function is not easy in procedural oriented programming. |
Problem Size | It is suitable for solving big problems. | It is not suitable for solving big problems. |
Data Access | In object oriented programming, data cannot move easily from function to function, it can be kept public or private so we can control the access of data. | In procedural oriented programming, most function uses global data for sharing that can be accessed freely from function to function in the system. |
Examples | Examples of object oriented programming languages include: C++, Java, VB.NET, C#.NET and Python. | C, VB, Fortran and Pascal are common examples of procedure oriented languages. |
READ FURTHER: Difference Between While And Do-While Loop In Java With Examples
Advantages Object-Oriented Programming
- OOP promotes code organization into modular, reusable components (objects/classes). This makes development more efficient and maintains a clean codebase.
- OOP’s modular nature makes it easier to locate and fix bugs or make updates. Changes made to one part of the code have less impact on other parts.
- OOP allows for easy addition of new features through inheritance and polymorphism. New classes can be created based on existing ones, minimizing code duplication.
- OOP enables developers to model real-world entities and their relationships more naturally, leading to more intuitive and readable code.
- OOP promotes team collaboration by allowing developers to work on different parts of a system independently, as long as the interfaces are well-defined.
Advantages of Procedural Programming
- Procedural programming can be simpler to understand, especially for small-scale programs or when the problem domain is straightforward.
- In some cases, procedural programs can be more efficient in terms of memory usage and execution speed, as they avoid the overhead of creating and managing objects.
- Procedural programs execute in a linear manner, making it easier to predict the sequence of actions and behavior.
- The step-by-step nature of procedural programming can be beneficial for tasks that involve clear, sequential processes.
Disadvantages of Procedural Programming
- Code reusability can be limited since functions are often closely tied to the specific program structure.
- Overuse of global variables can lead to issues with data integrity and make programs harder to debug and maintain.
- As programs grow larger and more complex, maintaining the control flow and managing global state can become challenging.
- Procedural programming might become less intuitive when dealing with complex interactions between data and behavior.