Abstract Class
An abstract class is a class that is declared abstract — it may or may not include abstract methods. Abstract classes cannot be instantiated, but they can be subclassed. An abstract class may have static fields and static methods. When an abstract class is subclassed, the subclass usually provides implementations for all of the abstract methods in its parent class. However, if it does not, then the subclass must also be declared abstract. An abstract method is a method that is declared without an implementation (without braces and followed by a semicolon).
Abstract Class Example
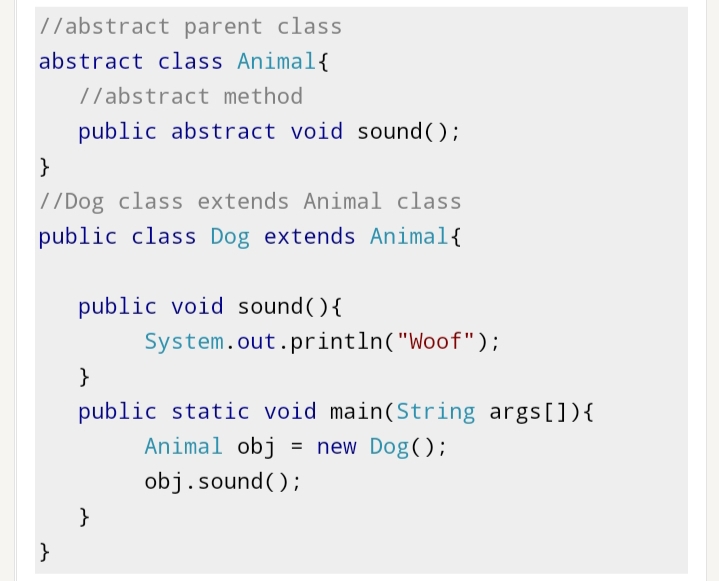
When To Use
Consider using abstract classes if any of these statements apply to your circumstance:
- You want to share code among several closely related classes.
- You expect that classes that extend your abstract class have many common methods or fields or require access modifiers other than public (such as protected and private).
- You want to declare non-static or non-final fields. This enables you to define methods that can access and modify the state of the object to which they belong.
What You Need To Know About Abstract Class
- An abstract class defines the identity of a class.
- Abstract class is fast.
- Abstract class can have both an abstract as well as concrete methods.
- Abstract class can have final, non-final, static and non-static variables.
- An abstract class can inherit a class and multiple interfaces.
- Abstract class is declared using abstract keyword.
- Abstract can have class members like private, public, protected etc.
- An abstract class can extend another Java class and implement multiple Java interfaces.
- An abstract can be extended using keyword ‘’extends.’’
- Abstract class can provide the implementation of interface.
- An abstract class can declare constructors and destructors.
- In an abstract class, the abstract keyword is compulsory for declaring a method as an abstract.
- Abstract class should be used when various implementations of the same kind share a common behavior.
- Abstract class can have an access modifier.
Interface
An interface is just the declaration of methods of an object; it’s not the implementation. In an interface, we define what kind of operation an object can perform. These operations are defined by the classes that implement the interface. Interfaces form a contract between the class and the outside world, and this contract is enforced at build time by the compiler.
Example Of Interface
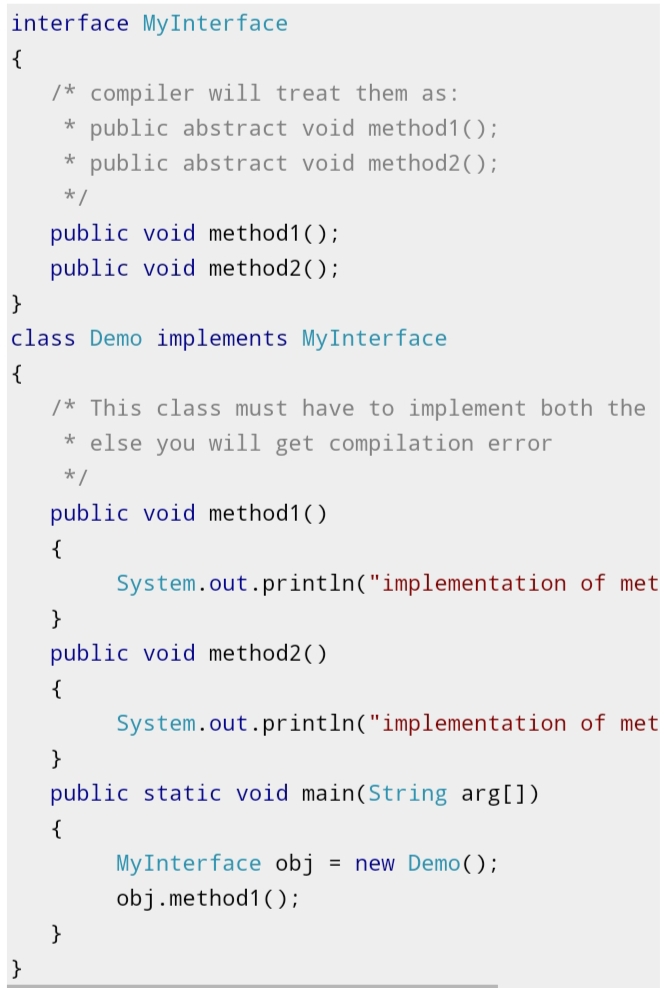
When To Use
Consider using interfaces if any of these statements apply to your circumstance:
- You expect that unrelated classes would implement your interface. For example, the interfaces Comparable and Cloneable are implemented by many unrelated classes.
- You want to specify the behavior of a particular data type, but not concerned about who implements its behavior.
- You want to take advantage of multiple inheritances.
What You Need To Know About Interface
- Interfaces help to define the peripheral abilities of a class.
- Interface is slow.
- Interface can have only abstract methods. Java 8 onwards, it can have default as well as static methods.
- Interface has only static and final variables.
- An interface can inherit multiple interfaces but cannot inherit a class.
- Interface is declared using interface keyword.
- Members of a Java interface are public by default.
- An interface can extend another Java interface only.
- An interface can be implemented using keyword ‘’implements.’’
- Interface can’t provide the implementation of abstract class.
- An interface cannot declare constructors and destructors.
- In an abstract, interface keyword is optional for declaring a method as an abstract.
- Interface is used when various implementations share only method signature. Polymorphic hierarchy of value types.
- The interface does not have access modifiers. Everything defined inside the interface is assumed public modifier.
Also Read: Difference Between Throw And Throws In Java
Difference Between Abstract Class And Interface In Tabular Form
BASIS OF COMPARISON | ABSTRACT CLASS | INTERFACE |
Function | An abstract class defines the identity of a class. | Interfaces help to define the peripheral abilities of a class. |
Speed | Abstract class is fast. | Interface is slow. |
Methods | Abstract class can have both an abstract as well as concrete methods. | Interface can have only abstract methods. Java 8 onwards, it can have default as well as static methods. |
Variables | Abstract class can have final, non-final, static and non-static variables. | Interface has only static and final variables. |
Inheritance | An abstract class can inherit a class and multiple interfaces. | An interface can inherit multiple interfaces but cannot inherit a class. |
Keyword | Abstract class is declared using abstract keyword. | Interface is declared using interface keyword. |
Members | Abstract can have class members like private, public, protected etc. | Members of a Java interface are public by default. |
Implementation | An abstract class can extend another Java class and implement multiple Java interfaces. | An interface can extend another Java interface only. |
Implementation Keyword | An abstract can be extended using keyword ‘’extends.’’ | An interface can be implemented using keyword ‘’implements.’’ |
Flexibility | Abstract class can provide the implementation of interface. | Interface can’t provide the implementation of abstract class. |
Constructors & Destructors | An abstract class can declare constructors and destructors. | An interface cannot declare constructors and destructors. |
Abstract Keyword | In an abstract class, the abstract keyword is compulsory for declaring a method as an abstract. | In an abstract, interface keyword is optional for declaring a method as an abstract. |
Usage | Abstract class should be used when various implementations of the same kind share a common behavior. | Interface is used when various implementations share only method signature. Polymorphic hierarchy of value types. |
Access Modifier | Abstract class can have an access modifier. | The interface does not have access modifiers. Everything defined inside the interface is assumed public modifier. |
Also Read: Difference Between Iterator And Enumeration