What Is For Loop?
A For-loop is a control flow statement for specifying iteration, which allows code to be executed repeatedly. A for-loop has two parts: a header specifying the iteration and a body which is executed once per iteration. The header often declares an explicit loop counter or loop variable, which allows the body to know which iteration is being executed. For-loops are typically used when the number of iterations is known before entering the loop. For-loops can be thought of as shorthands for while-loops which increment and test a loop variable.
The “for” loop provides a convenient and concise way to specify the initialization, condition, and update steps for the loop.
Here’s the basic syntax of a “for” loop in Java:
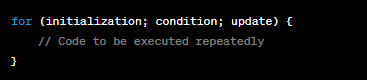
Let’s break down each part of the “for” loop:
- Initialization: This is where you initialize a loop control variable. It typically serves as a counter or an iterator. The initialization is executed only once at the beginning of the loop.
- Condition: This is a boolean expression that determines whether the loop should continue or terminate. If the condition evaluates to true, the loop body is executed; otherwise, the loop terminates.
- Update: This part is responsible for modifying the loop control variable after each iteration. It is executed at the end of each iteration, just before the condition is re-evaluated.
Here’s an example of a simple “for” loop that counts from 1 to 5:
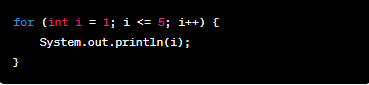
In this example:
- The initialization sets
int i
to 1. - The condition checks if
i
is less than or equal to 5. - The update increments
i
by 1 (i++
) after each iteration. - The loop prints the value of
i
in each iteration, resulting in the numbers 1 through 5 being printed.
What You Need To Know About For Loop
- The for loop is a control structure for specifying iteration that allow code to be repeatedly executed.
- It uses an index of an element to fetch data from an array.
- Termination of this loop is controlled by loop condition. Therefore, For loop executes repeatedly until the given condition turns out to be false.
- It requires loop counter, initial and end values, in order to iterate through all the elements of an array.
- It was introduced in Java 1.
- It works on indexable objects where random access of elements is allowed.
- When we iterate through using traditional for loop we can manipulate the actual data of an array.
- The For loop is harder to read and write than the Foreach loop.
- A for loop can be used to retrieve a particular set of elements.
What Is For-Each Loop?
Foreach loop is a control flow statement for traversing items in a collection. Foreach is usually used in place of a standard for loop statement. Unlike other for loop constructs, however, foreach loops usually maintain no explicit counter: they essentially say “do this to everything in this set”, rather than “do this x times”. This avoids potential off-by-one errors and makes code simpler to read. In object-oriented languages an iterator, even if implicit, is often used as the means of traversal.
The foreach statement in some languages has some defined order, processing each item in the collection from the first to the last. The foreach statement in many other languages, especially array programming languages, does not have any particular order. This simplifies loop optimization in general and in particular allows vector processing of items in the collection concurrently.
In Java, the “for-each” loop is known as the “enhanced for loop” or “foreach loop.”
Syntax: The syntax of the enhanced for loop in Java is straightforward:
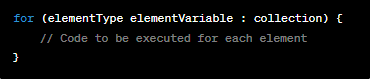
Here’s a breakdown of the components:
elementType
: This is the data type of the elements in the collection. It should match the type of elements in the collection.elementVariable
: This is a variable that represents each individual element in the collection as the loop iterates. You declare and initialize this variable within the loop.collection
: This is the collection of items you want to iterate over. It must be an array, collection, or any object that implements theIterable
interface.- The code block inside the loop is the set of statements that are executed for each element in the collection.
How it works:
- The enhanced for loop initializes the
elementVariable
with the first element in thecollection
. - It then executes the code block inside the loop, which operates on the
elementVariable
. - After completing the code block for the first element, the loop automatically moves to the next element in the collection.
- Steps 2 and 3 repeat until all elements in the collection have been processed.
Advantages:
- The enhanced for loop simplifies code by abstracting away the loop control logic and providing a clean, readable syntax.
- It helps prevent common loop-related errors, such as index-out-of-bounds errors, making it safer to use.
- The code written using the enhanced for loop is typically more concise than equivalent code written with a traditional “for” loop.
Limitations
- The enhanced for loop is read-only, meaning you cannot modify the elements of the collection while iterating. Attempting to do so will result in a compilation error.
- You cannot directly access the index of the current element within the enhanced for loop. If you need the index, you may need to use a traditional “for” loop instead.
- It is best suited for sequential iteration through all elements of a collection. If you need more complex control, such as skipping elements or iterating in reverse, you may need a traditional loop.
What You Need To Know About For-Each Loop
- The foreach loop is a control structure for traversing items in an array or a collection.
- It uses an iteration variable to automatically fetch data from an array.
- Break statement can be used to terminate the execution of the loop early, otherwise, it executes until the last element gets evaluated.
- It automates the iteration by using the iteration variable which stores one element each time.
- It was introduced back in Java 5.
- It works on all indexable objects even when random access of an individual element is not allowed.
- The iteration variable in for-reach is read-only. It means changes made on iteration value would not affect the actual data of the collection or array.
- The Foreach loop is easier to read and write than the for loop.
- The Foreach loop cannot be used to retrieve a particular set of elements.
For vs For –Each Loop: Key Differences
Aspect | for Loop | for-each Loop |
---|---|---|
Syntax | Uses a traditional syntax with initialization, condition, and iteration expressions. | Uses an enhanced for-each syntax with an iterable object. |
Iterable Objects | Suitable for arrays, collections, and other iterable objects. | Specifically designed for iterating through arrays and collections. |
Element Access | Requires explicit indexing or element retrieval. | Automatically accesses each element without explicit indexing. |
Loop Control | Provides fine-grained control over the loop process with the ability to skip iterations, break, or continue. | Limited control; doesn’t support skipping or modifying elements during iteration. |
Index Access | Can access elements by index. | Doesn’t provide direct access to index values. |
Modification | Allows modifying elements within the loop body. | Typically used for read-only access; modifying elements is generally not supported. |
Null Values | Can handle null elements within the iterable object. | May throw a NullPointerException if used with a null iterable. |
Performance | Slightly more efficient for indexed access or complex looping logic. | Generally simpler and cleaner code, but may be less efficient for certain use cases. |
Use Cases | Suitable for various looping scenarios, especially when you need control over the index and want to modify elements. | Preferred for simple iteration over collections or arrays where modification isn’t required. |
Code Clarity | Often requires more lines of code, making it potentially less readable for simple iterations. | Results in concise and more readable code for straightforward iteration tasks. |